Array in string umwandeln c?
Hallo,
ich habe ein Hex Array mit beliebigen Inhalten.Ich möchte diesen Inhalt nun in eine string packen. Wie könnte ich das in C lösen?
Bsp:
array[0] = 05
array[1] = AE
-> string = 05AE
4 Antworten
Vom Fragesteller als hilfreich ausgezeichnet
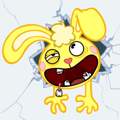
include <stdio.h>
int *a, *b;
void f(int x) {
int i[3];
i[0] = x;
i[1] = x + 1;
i[2] = x + 2;
a = i;
}
void g(int x) {
int i[3];
i[0] = x;
i[1] = x + 1;
i[2] = x + 2;
b = i;
}
int main() {
f(1);
printf("a = {%d,%d,%d}\n", a[0], a[1], a[2]);
g(2);
printf("a = {%d,%d,%d}\n", a[0], a[1], a[2]);
}
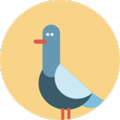
#include <stdio.h> /* fputs, puts */
#include <stdlib.h> /* calloc, EXIT_FAILURE, ... */
#include <stddef.h> /* size_t */
char * hexstr(
const unsigned char * const buf,
const size_t len
) {
static const char * const hex = "0123456789ABCDEF";
char * const str = calloc(len + 1, sizeof(* str));
if (str) {
for (size_t i = 0; i < len; ++i) {
const unsigned char c = buf[i];
str[i * 2] = hex[c >> 4];
str[i * 2 + 1] = hex[c & 0xF];
}
}
return str;
}
int main(void) {
int result = EXIT_FAILURE;
const unsigned char buf[] = { 0x23, 0x42, 0xBE, 0xEF };
const size_t len = sizeof(buf) / sizeof(* buf);
char * const str = hexstr(buf, len);
if (str) {
puts(str);
free(str);
result = EXIT_SUCCESS;
} else {
fputs("ERROR: Memory allocation failed.", stderr);
}
return result;
}
Woher ich das weiß:Berufserfahrung
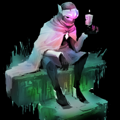
Hallo, in C gibt es keine Strings sonder nur Char-Pointer/Char-Arrays.
Einfach ein Char-Array mit genügend Platz erstellen und die Character aus den Originalarrays hintereinander reinkopieren.
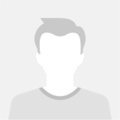
sowas?
http://www.c-howto.de/tutorial/strings-zeichenketten/string-funktionen/strings-verketten/
oder in C++ die Merge Function
Woher ich das weiß:Studium / Ausbildung